Elastic workflow#
This is a elastic workflow that is based on a stress-strain approach.
If you want to read more about this approach, please check out https://docs.materialsproject.org/methodology/materials-methodology/elasticity and the following publication https://www.nature.com/articles/sdata20159.
Let’s install atomate2 first.
%%capture
!pip install atomate2[strict]
Let’s start with the workflow#
We now simply load the force field elastic workflow. We change from the default universal ML potential to M3GNet. For CHGNet, an updated version of the ML potential needs to be used: CederGroupHub/chgnet#79
from pymatgen.core import Structure
from pymatgen.symmetry.analyzer import SpacegroupAnalyzer
from atomate2.forcefields.flows.elastic import ElasticMaker
from atomate2.forcefields.jobs import M3GNetRelaxMaker
structure = Structure(
lattice=[[0, 2.73, 2.73], [2.73, 0, 2.73], [2.73, 2.73, 0]],
species=["Si", "Si"],
coords=[[0, 0, 0], [0.25, 0.25, 0.25]],
)
structure= SpacegroupAnalyzer(structure).get_primitive_standard_structure()
elastic_flow = ElasticMaker(
bulk_relax_maker=M3GNetRelaxMaker(
relax_cell=True, relax_kwargs={"fmax": 0.00001}
),
elastic_relax_maker=M3GNetRelaxMaker(
relax_cell=False, relax_kwargs={"fmax": 0.00001}
),
).make(structure)
/home/jgeorge/miniconda3/envs/pythonProject1/lib/python3.11/site-packages/tqdm/auto.py:21: TqdmWarning: IProgress not found. Please update jupyter and ipywidgets. See https://ipywidgets.readthedocs.io/en/stable/user_install.html
from .autonotebook import tqdm as notebook_tqdm
/home/jgeorge/miniconda3/envs/pythonProject1/lib/python3.11/site-packages/spglib/spglib.py:115: DeprecationWarning: dict interface (SpglibDataset['number']) is deprecated.Use attribute interface ({self.__class__.__name__}.{key}) instead
warnings.warn(
/home/jgeorge/miniconda3/envs/pythonProject1/lib/python3.11/site-packages/spglib/spglib.py:115: DeprecationWarning: dict interface (SpglibDataset['international']) is deprecated.Use attribute interface ({self.__class__.__name__}.{key}) instead
warnings.warn(
Let’s have a look at the computing jobs#
The phonon run will first perform a bulk relaxation, then the displacements are generated and run. As we currently don’t have a way to compute BORN charges with such potentials, a non-analytical term correction is not performed here.
elastic_flow.draw_graph().show()
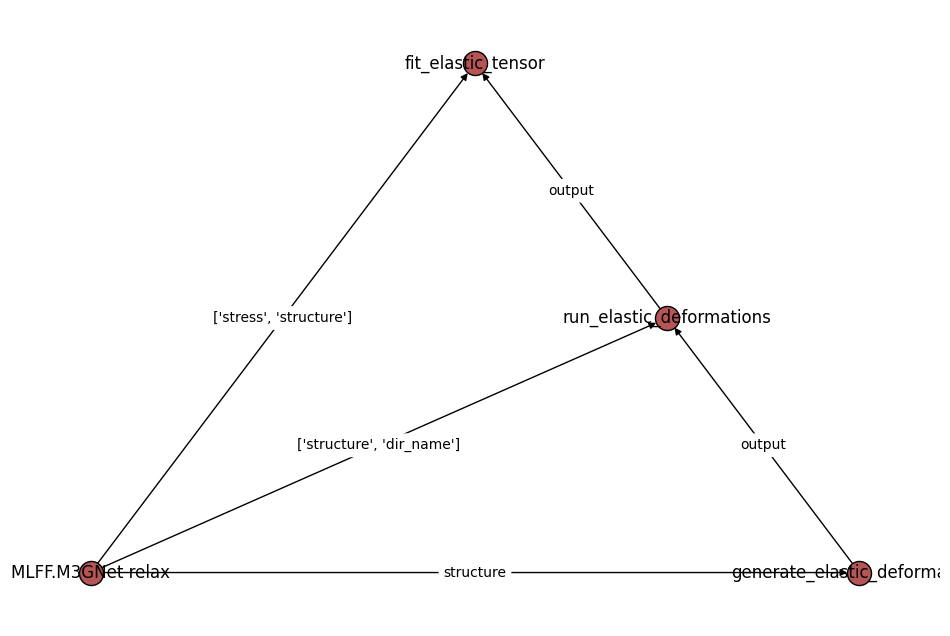
Let’s execute the workflow#
%%capture
from jobflow import run_locally
responses = run_locally(elastic_flow, create_folders=True)
Let’s have a look at the outputs#
We query our database for the relevant outputs (here results from the elastic document)
from jobflow import SETTINGS
store = SETTINGS.JOB_STORE
store.connect()
result = store.query_one(
{"name": "fit_elastic_tensor"},
properties=[
"output.elastic_tensor",
"output.derived_properties",
],
load=True,
sort={"completed_at": -1} # to get the latest computation
)
You can then access the elastic tensor in different output formats:
print(result["output"]["elastic_tensor"]["ieee_format"])
[[1964044.7930349563, 80.13000784632267, 80.13003478218025, -3.983617891299374e-06, -7.274317331366699e-06, 3.4037173399407686e-05], [80.13000784632264, 1964042.4957294029, 80.12998791559116, -5.617618620915702e-06, -5.158386258075467e-06, 0.5916081580179327], [80.13003478218025, 80.12998791559117, 1964043.8244994986, -0.09764127632641656, -0.12643575730690002, 2.414301115201581e-05], [-3.983617891322431e-06, -5.6176186209065425e-06, -0.09764127632641659, 16.434144813096356, 4.956572730741239e-06, -1.0579531677767372e-06], [-7.274317331391944e-06, -5.158386258084771e-06, -0.12643575730690004, 4.956572730741188e-06, 16.434154427743533, -8.170161332630009e-07], [3.403717339939713e-05, 0.5916081580179325, 2.414301115202968e-05, -1.0579531677767368e-06, -8.170161332629465e-07, 16.434149038558008]]
And, derived properties such as bulk moduli can be accessed:
print(result["output"]["derived_properties"])
{'k_voigt': 654734.6548138828, 'k_reuss': 654734.6522743432, 'k_vrh': 654734.653544113, 'g_voigt': 392802.57537187694, 'g_reuss': 27.389943344694814, 'g_vrh': 196414.98265761082, 'universal_anisotropy': 71700.62027613331, 'homogeneous_poisson': 0.3636397802114065, 'y_mod': 535678567562903.2, 'trans_v': 292464.44905979116, 'long_v': 631801.6381676111, 'snyder_ac': 42554919.35786479, 'snyder_opt': 18.76156133557427, 'snyder_total': 42554938.119426124, 'clark_thermalcond': None, 'cahill_thermalcond': 90.99458211314227, 'debye_temperature': 35947.944080301946}